Sometime user would like to repeat order but they contacted you manually. In this case, the fastest way to submit the order on their behalf is to find existing order, and convert them to customer.
Therefore, on new order, you could just search their email to submit order.
There are two parts to do this.
A script to convert existing guest to customer and then a script to auto register guest as customer.
Script to convert guest to customer
/** * Plugin Name: WooCommerce Convert Guest to Customer * Description: Adds a button to convert guest orders to customer accounts on the WooCommerce order details page. * Version: 1.0 * Author: Your Name */ if (!defined('ABSPATH')) { exit; // Exit if accessed directly } /** * Add "Convert to Customer" button for guest orders */ function add_convert_to_customer_button($order) { // Check if the order is by a guest user if ($order->get_user_id() === 0) { $nonce = wp_create_nonce('convert_guest_to_customer'); $order_id = $order->get_id(); echo '<button class="button" id="convert-to-customer" data-order-id="' . esc_attr($order_id) . '" data-nonce="' . esc_attr($nonce) . '">Convert to Customer</button>'; } } add_action('woocommerce_admin_order_data_after_billing_address', 'add_convert_to_customer_button', 10, 1); /** * Enqueue JavaScript for AJAX functionality */ function enqueue_convert_to_customer_script() { wp_enqueue_script('convert-to-customer', plugins_url('convert-to-customer.js', __FILE__), array('jquery'), '1.0', true); wp_localize_script('convert-to-customer', 'convert_to_customer_vars', array( 'ajax_url' => admin_url('admin-ajax.php') )); } add_action('admin_enqueue_scripts', 'enqueue_convert_to_customer_script'); /** * AJAX handler to convert guest to customer */ function convert_guest_to_customer() { check_ajax_referer('convert_guest_to_customer', 'nonce'); $order_id = isset($_POST['order_id']) ? intval($_POST['order_id']) : 0; $order = wc_get_order($order_id); if (!$order) { wp_send_json_error('Invalid order ID'); return; } $email = $order->get_billing_email(); $first_name = $order->get_billing_first_name(); $last_name = $order->get_billing_last_name(); // Check if user already exists $user = get_user_by('email', $email); if ($user) { wp_send_json_error('User already exists'); return; } // Create new user $username = sanitize_user(current(explode('@', $email)), true); $counter = 1; $new_username = $username; while (username_exists($new_username)) { $new_username = $username . $counter; $counter++; } $password = wp_generate_password(); $user_id = wp_create_user($new_username, $password, $email); if (is_wp_error($user_id)) { wp_send_json_error($user_id->get_error_message()); return; } // Update user meta update_user_meta($user_id, 'first_name', $first_name); update_user_meta($user_id, 'last_name', $last_name); // Update order with new user ID $order->set_customer_id($user_id); $order->save(); // Send email to the new user wp_new_user_notification($user_id, null, 'user'); wp_send_json_success('User created successfully'); } add_action('wp_ajax_convert_guest_to_customer', 'convert_guest_to_customer'); add_action('wp_ajax_nopriv_convert_guest_to_customer', 'convert_guest_to_customer'); /** * Add inline JavaScript */ function add_convert_to_customer_inline_script() { ?> <script type="text/javascript"> jQuery(document).ready(function($) { $('#convert-to-customer').on('click', function(e) { e.preventDefault(); var button = $(this); var order_id = button.data('order-id'); var nonce = button.data('nonce'); $.ajax({ url: convert_to_customer_vars.ajax_url, type: 'POST', data: { action: 'convert_guest_to_customer', order_id: order_id, nonce: nonce }, beforeSend: function() { button.prop('disabled', true).text('Converting...'); }, success: function(response) { if (response.success) { alert('Guest converted to customer successfully!'); button.remove(); } else { alert('Error: ' + response.data); } }, error: function() { alert('An error occurred. Please try again.'); }, complete: function() { button.prop('disabled', false).text('Convert to Customer'); } }); }); }); </script> <?php } add_action('admin_footer', 'add_convert_to_customer_inline_script');
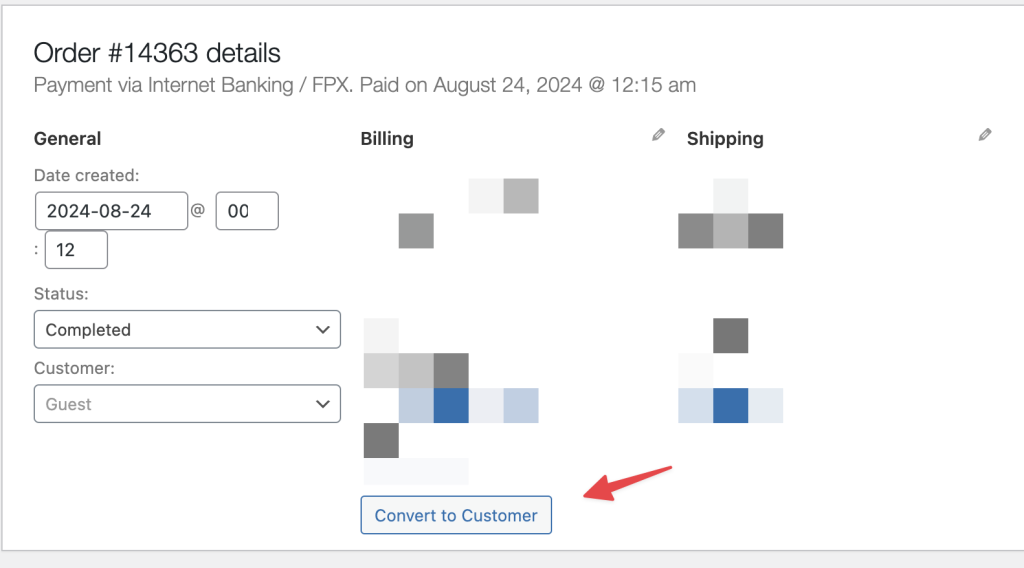
Script to auto register guest as customer
/** * @snippet Register Guest Users @ WooCommerce Checkout * @how-to Get CustomizeWoo.com FREE * @author Rodolfo Melogli * @testedwith WooCommerce 8 * @community https://businessbloomer.com/club/ */ add_action( 'woocommerce_thankyou', 'bbloomer_register_guests', 9999 ); function bbloomer_register_guests( $order_id ) { $order = wc_get_order( $order_id ); $email = $order->get_billing_email(); if ( ! email_exists( $email ) && ! username_exists( $email ) ) { $customer_id = wc_create_new_customer( $email, '', '', array( 'first_name' => $order->get_billing_first_name(), 'last_name' => $order->get_billing_last_name(), )); if ( is_wp_error( $customer_id ) ) { throw new Exception( $customer_id->get_error_message() ); } wc_update_new_customer_past_orders( $customer_id ); wc_set_customer_auth_cookie( $customer_id ); } else { $user = get_user_by( 'email', $email ); wc_update_new_customer_past_orders( $user->ID ); } }
You can use Code Snippet plugin to do this.